Word Game in Python: A Scrabble-Inspired Letter Game
The Word Game is a fun and interactive project that combines vocabulary skills with strategy.
Project Title and Overview
Title:
Word Game in Python: A Scrabble-Inspired Letter Game
Overview:
This project implements a word creation game in Python, inspired by Scrabble. The player is dealt a random set of letters, including vowels and consonants, and must use them to form words. Each word is scored based on letter values (similar to Scrabble), and bonus points are awarded for using all the letters in a single hand. The player can play multiple rounds, replay hands, or end the game.
Project Objective
Objective:
The objective of the game is to create the highest-scoring words using the letters in the player's hand. The game rewards the player for creating longer words and for using all the letters in their hand.
Key Use Cases:
- Educational: A fun way for kids and word enthusiasts to practice vocabulary and spelling.
- Entertainment: A simple game for anyone looking to have fun with word creation, inspired by Scrabble.
Technology Stack
Libraries:
- Python Standard Libraries:
- random: Used to shuffle and distribute letters to the player.
- string: For handling available letters (a-z).
- input/output: Basic I/O for user interaction.
Game Logic and Rules
Word Scoring:
Each letter has a Scrabble-inspired point value. The player’s score is calculated by summing the points for each letter in their word, multiplied by the word's length. A 50-point bonus is awarded if the player uses all their letters in one word.
def getWordScore(word, n):
score = 0
for i in word:
score += SCRABBLE_LETTER_VALUES[i]
score *= len(word)
if len(word) == n:
score += 50
return score
Game Rounds:
- The player is dealt a hand of random letters (vowels and consonants).
- They can enter a word or a single period (
"."
) to end the hand. - The game validates whether the word is:
- Present in the word list.
- Composed only of letters from the current hand.
- After each valid word, the hand is updated, and the player receives feedback on their score.
- The hand ends when all letters are used or the player opts to finish the hand.
Hand Display:
The hand is displayed to the player in a format that shows the available letters for word creation.
def displayHand(hand):
for letter in hand.keys():
for j in range(hand[letter]):
print(letter, end=" ") # prints letters in a readable format
print()
Important Functions
Word Validation:
The game checks whether the player’s word is valid by ensuring that:
- The word exists in the preloaded word list.
- The word uses only letters available in the current hand.
def isValidWord(word, hand, wordList):
hand_copy = hand.copy()
if word not in wordList:
return False
for letter in word:
if hand_copy.get(letter, 0) == 0:
return False
hand_copy[letter] -= 1
return True
Hand Management:
- Updating the Hand: Once the player uses a letter, it is removed from their hand.
def updateHand(hand, word):
hand_copy = hand.copy()
for letter in word:
hand_copy[letter] -= 1
return hand_copy
Key Challenges
Challenges Faced:
- Validating Words: Ensuring that the game correctly checks if a word is composed of letters from the current hand.
- Scoring System: Accurately implementing the scoring system to reward longer words and full-hand usage.
Achievements and Future Work
Achievements:
- Successfully implemented a word-building game that is easy to play and engaging.
- Introduced replay and multiple rounds, allowing users to play with new or repeated hands.
- Implemented a scoring system inspired by Scrabble, which encourages strategic word creation.
Future Work:
- Graphical Interface: Develop a GUI using Pygame or Tkinter to make the game visually interactive.
- Multiplayer Mode: Add multiplayer functionality where two players compete to create the highest-scoring words.
- Difficulty Levels: Introduce different levels of difficulty by adjusting hand sizes or word requirements.
Results
- Game Start:
- The player is dealt a random set of letters (hand), and the game prompts them to create a word.
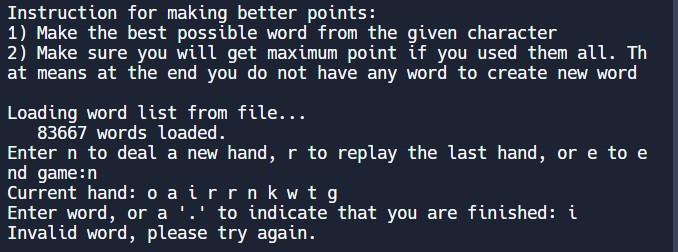
- Word Entry and Scoring:
- After each valid word, the score for the word and the remaining letters are displayed.
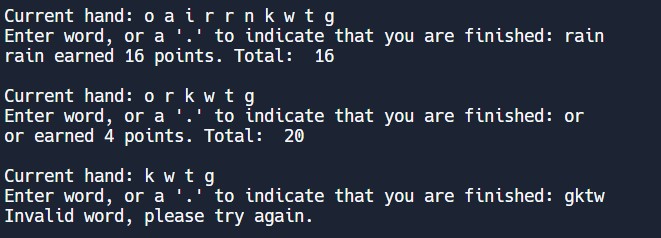
- End of Hand:
- The game displays the final score for the hand and allows the player to replay or start a new hand.

Conclusion
The Word Game is a fun and interactive project that combines vocabulary skills with strategy. By implementing Scrabble-style scoring, it encourages players to maximize their points with each word. This project showcases how Python can be used to create engaging and educational games.