Project Title and Overview
Title:
Hangman Game in Python: A Fun Word-Guessing Game
Overview:
This project is a simple and interactive Hangman game built in Python. The game randomly selects a secret word from a pre-loaded word list, and the player must guess the word by suggesting letters within a limited number of attempts. The game provides feedback after every guess, displaying the correctly guessed letters and the remaining available letters.
Project Objective
Objective:
The main objective of this project is to create an enjoyable, console-based word-guessing game where the user attempts to guess a secret word. The user has a limited number of wrong guesses (8 chances), and the game tracks their progress by showing the partially guessed word and remaining letters.
Key Use Cases:
- Educational: A great way for kids and beginners to learn new words and practice spelling.
- Fun & Entertainment: Simple entertainment for users of all ages, challenging their word knowledge.
Technology Stack
Libraries:
- Python Standard Libraries:
- random: Used for selecting a random word from the word list.
- string: For handling the available letters (a-z).
- input/output: Basic I/O functions for user interaction.
Game Logic and Rules
Word Selection:
The game loads a list of words from a file, words.txt
, and randomly selects one word as the secret word. The length of the word is displayed to the user to help them start guessing.
# Load the list of words and select a random word
wordlist = loadWords()
secretWord = chooseWord(wordlist).lower()
Gameplay:
- User Input: The player inputs one letter at a time.
- Correct Guess: If the guessed letter is in the word, the player is informed, and the correctly guessed letters are shown in their positions.
- Wrong Guess: If the letter is not in the word, the player loses one guess, and the number of remaining guesses is displayed.
- Repetitions: If the player guesses a letter that they already guessed, the game informs them without penalizing their remaining guesses.
End Conditions:
- The game ends when:
- Player Wins: The player correctly guesses the word within the allowed guesses.
- Player Loses: The player runs out of guesses before completing the word.
if isWordGuessed(secretWord, lettersGuessed):
print('Congratulations, you won!')
else:
print('Sorry, you ran out of guesses. The word was', secretWord)
Important Functions
Word Guessing Logic:
isWordGuessed(secretWord, lettersGuessed)
: Checks if the player has guessed all the letters in the secret word.getGuessedWord(secretWord, lettersGuessed)
: Returns the current state of the guessed word, with correctly guessed letters and underscores for the remaining letters.getAvailableLetters(lettersGuessed)
: Displays the letters that the player hasn’t guessed yet.
def getGuessedWord(secretWord, lettersGuessed):
result = ''
for i in secretWord:
if i in lettersGuessed:
result += i
else:
result += '_ '
return result
User Interaction:
The game continuously prompts the player for guesses, informs them if the guess was correct or incorrect, and displays their progress and remaining available letters after each round.
print('You have', guessesLeft, 'guesses left.')
print('Available Letters:', getAvailableLetters(lettersGuessed))
guess = input('Please guess a letter: ').lower()
Key Challenges
Challenges Faced:
- User Input Handling: Managing user input (correct, incorrect, repeated guesses) without causing confusion.
- Game Feedback: Providing real-time feedback on the guessed letters and available letters to improve user experience.
Achievements and Future Work
Achievements:
- Successfully created an interactive Hangman game that provides real-time feedback to users.
- Implemented a feature to display available letters to help users track their guesses.
- Random word generation ensures the game remains fresh and challenging every time.
Future Work:
- Graphical Interface: Convert the console-based game into a GUI using libraries like Pygame or Tkinter.
- Multiplayer Mode: Add a feature where two players can compete to guess words.
Results
Here are some example screenshots showing the game in progress: Find code here
- Start of the Game:
- The secret word is chosen, and the number of letters is displayed to the user.

- User Guessing:
- The user guesses a letter, and the game updates the guessed word and available letters.

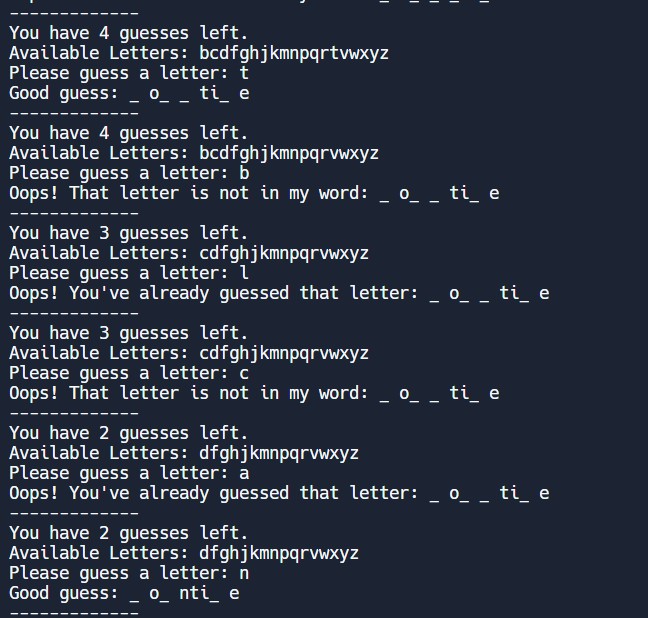
- End of the Game:
- The user either wins by guessing the word or loses if they run out of guesses.
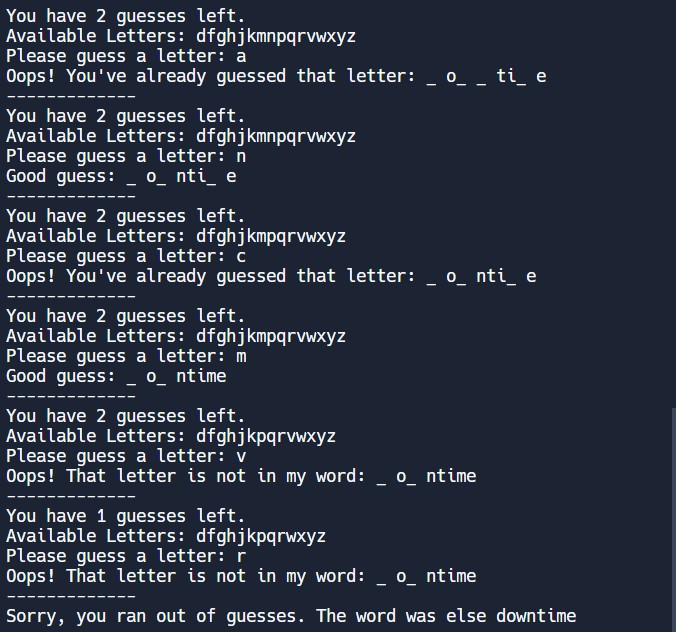
Conclusion
This Hangman Game is a simple yet engaging project that showcases fundamental programming concepts such as string manipulation, loops, conditionals, and user interaction. It offers a great learning opportunity for Python beginners and a fun way to test vocabulary skills.